Deleting records in ASP.NET Core using AJAX(jQuery Unobtrusive Ajax library)
Published: 14 August 2018
Now, let’s look at how we can improve the experience using Ajax and the jQuery Unobtrusive Ajax library.
First, you will need to add the jQuery Unobtrusive Ajax library to your project. I downloaded the JavaScript file, added it to my project and referenced it in my _Layout
view.
Next, we’ll need to update the <form>
tag we declared in the View which calls the Delete
handler to add a data-ajax
attribute. This tells jQuery Unobtrusive to intercept that postback and make an Ajax call instead.
<form asp-action="Delete" asp-route-id="@customer.Id" data-ajax="true">
<button type="submit" class="btn btn-sm btn-danger d-none d-md-inline-block">
Delete
</button>
</form>
We will also need to update Delete
action. Previously, it redirected the user to the Index
action after a record was deleted. Since this action is now invoked using Ajax, we do not have to do the redirect anymore - or return any content for that matter.
[HttpPost]
public IActionResult Delete(int id)
{
_database.Customers.RemoveAll(customer => customer.Id == id);
return NoContent();
}
At this point, the customer will be deleted using Ajax, but the list of customers will not be updated to reflect the fact that the customer was deleted. To do this, we will need to manually delete the <li>
element containing the customer information after the Ajax call has completed.
We need some sort of callback after the Ajax call has completed so we can handle this deletion. The jQuery Unobtrusive Ajax library allows us to do this by specifying a function to be called using the data-ajax-success
attribute.
Let’s update the <form>
to add this attribute which specifies a deleteItem
function, passing the form element itself as a parameter:
<form asp-action="Delete" asp-route-id="@customer.Id" data-ajax="true" data-ajax-success="deleteItem(this)">
<button type="submit" class="btn btn-sm btn-danger d-none d-md-inline-block">
Delete
</button>
</form>
The delete item itself is pretty straight-forward. We traverse the DOM tree for the ancestors of the <form>
and look for that <li>
parent element. Once we find it, we remove it:
<script>
function deleteItem(form) {
$(form).parents('li').remove();
}
</script>
With that in place, here is another animation demonstrating this improved version. Notice the network traffic in the developer tools window which indicates the requests initiated by clicking the Delete button as XHR requests (i.e. Ajax calls):
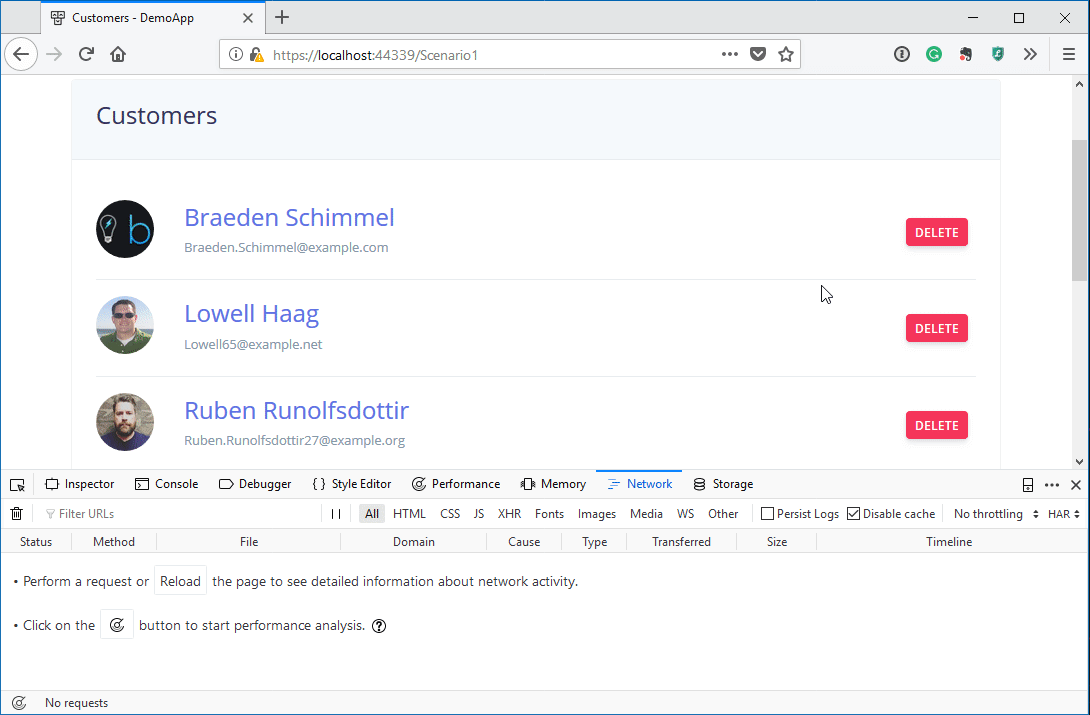
Conclusion
In this blog post, I showed how you could create a better user experience using Ajax to delete a customer record. It is a relatively simple process which can be added to an existing code base and does not require you to go all-in on any of the modern JavaScript frameworks.
Source code is available at https://github.com/jerriepelser-blog/aspnetcore-ajax. Have a look at the Scenario 1 controller and views.