Introduction
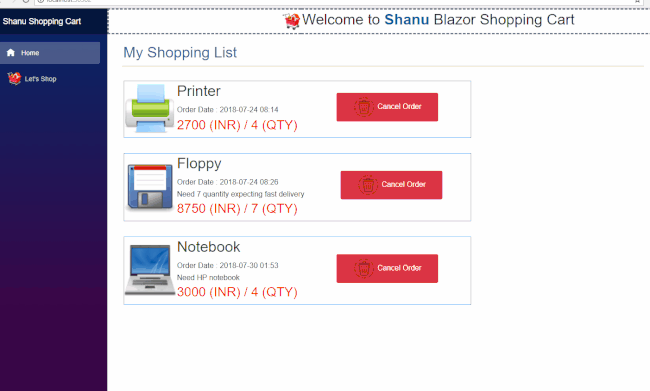
In this article, let’s see how to create our own ASP.NET Core Blazor Shopping Cart using Entity Framework, and Web API. Kindly read my previous articles which explain in depth about getting started with ASP.NET Core Blazor.
- ASP.NET Core Blazor CRUD Using Entity Framework And Web API
- ASP.NET Core Blazor Filtering And Sorting Using Entity Framework And Web API
- ASP.NET Core Blazor Master Detail Grid With Filtering And Sorting Using EF And Web API
- ASP.NET Core Blazor Master/Detail CRUD With Filtering And Sorting Using EF And Web API
This article will explain:
- Creating a sample database with ItemDetails and ShoppingDetails table in SQL Server to display in our web application.
- Creating ASP.NET Core Blazor Application.
- Creating EF, DBContext Class, and Model Class in Blazor Shared project.
- Creating WEB API in Blazor Server project.
- Creating Razor page for displaying Item details to add items to cart.
- Filtering Items by Item Name. From Item textbox, keyup event displays the items by search name.
- Selecting and adding items to the shopping cart.
- Saving the selected shopping item in the database.
- Displaying My Shopping Order Item details.
- Deleting the Ordered Items.
In this shopping cart demo application, we have 3 parts.
- Display all items and filter items in HTML Table using ASP.NET Core Blazor from Web API.
- Add Items to the shopping cart and Save the shopped items to the database.
- Show the user Shopping Order Items in the home page.
Display All Items and Filter Items
First, we display all item details in the shopping page using ASP.NET Core Blazor. All the item details will be loaded from Web API results and bound in Razor page. The user can also filter the items by "Item Name". When users enter any character in the "Item Name" Filter textbox, the related item details will be loaded dynamically from the database to the shopping page.

Add Items to shopping cart
When the user clicks on "Image name", we display the item details at the top, to add the selected item to shopping cart. The user can add or reduce the item quantity from the selected shopping cart. The user can save the selected shopping item to the database. Here we will not focus on the user login part and we have fixed one user as “Shanu” by default.

Shopping Order Items
In the home page, we display the user shopped order details with Item Image, Item Name, shopping description, shopped quantity, shopped amount and with delete order options.

Prerequisites
Make sure you have installed all the prerequisites in your computer. If not, then download and install all, one by one. Note that since Blazor is the new framework, we must have installed the preview of Visual Studio 2017 (15.7) or above.
- Install the .NET Core 2.1 Preview 2 SDK. (You can find all versions from this link
- Install the latest preview of Visual Studio 2017 (15.7).
- Install the NET Core Blazor Language Services for Blazor Extensions.
Code part
Step 1 - Create a database and a table
We will be using our SQL Server database for our WEB API and EF. First, we will create a database named ShoppingDB and a table as ItemDetails and ShoppingDetails. Here is the SQL script to create a database table and sample record insert query in our table. Run the query given below in your local SQL Server to create a database and a table to be used in our project.
- -- =============================================
- -- Author : Shanu
- -- Create date : 2017-02-03
- -- Description : To Create Database,Table and Sample Insert Query
- -- Latest
- -- Modifier : Shanu
- -- Modify date : 2017-02-03
- -- =============================================
- --Script to create DB,Table and sample Insert data
- USE MASTER
- GO
- -- 1) Check for the Database Exists .If the database is exist then drop and create new DB
- IF EXISTS (SELECT [name] FROM sys.databases WHERE [name] = 'ShoppingDB' )
- DROP DATABASE ShoppingDB
- GO
- CREATE DATABASE ShoppingDB
- GO
- USE ShoppingDB
- GO
- -- 1) //////////// ItemDetails table
- -- Create Table ItemDetails,This table will be used to store the details like Item Information
- CREATE TABLE ItemDetails
- (
- Item_ID int identity(1,1),
- Item_Name VARCHAR(100) NOT NULL,
- Item_Price int NOT NULL,
- Image_Name VARCHAR(100) NOT NULL,
- Description VARCHAR(100) NOT NULL,
- AddedBy VARCHAR(100) NOT NULL,
- CONSTRAINT [PK_ItemDetails] PRIMARY KEY CLUSTERED
- (
- [Item_ID] ASC
- )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
- ) ON [PRIMARY]
- GO
- -- Insert the sample records to the ItemDetails Table
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Access Point',950,'Images/AccessPoint.png','Access Point for Wifi use','Shanu')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('CD',350,'Images/CD.png','Compact Disk','Afraz')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Desktop Computer',1400,'Images/DesktopComputer.png','Desktop Computer','Shanu')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('DVD',1390,'Images/DVD.png','Digital Versatile Disc','Raj')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('DVD Player',450,'Images/DVDPlayer.png','DVD Player','Afraz')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Floppy',1250,'Images/Floppy.png','Floppy','Mak')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('HDD',950,'Images/HDD.png','Hard Disk','Albert')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('MobilePhone',1150,'Images/MobilePhone.png','Mobile Phone','Gowri')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Mouse',399,'Images/Mouse.png','Mouse','Afraz')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('MP3 Player ',897,'Images/MultimediaPlayer.png','Multi MediaPlayer','Shanu')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Notebook',750,'Images/Notebook.png','Notebook','Shanu')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Printer',675,'Images/Printer.png','Printer','Kim')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('RAM',1950,'Images/RAM.png','Random Access Memory','Jack')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('Smart Phone',679,'Images/SmartPhone.png','Smart Phone','Lee')
- Insert into ItemDetails(Item_Name,Item_Price,Image_Name,Description,AddedBy) values('USB',950,'Images/USB.png','USB','Shanu')
- select * from ItemDetails
- -- 2) //////////// ShoppingDetails table
- -- Create Table ShoppingDetails,This table will be used to store the user shopped items
- CREATE TABLE ShoppingDetails
- (
- shopId int identity(1,1),
- Item_ID int ,
- UserName VARCHAR(100) NOT NULL,
- Qty int NOT NULL,
- TotalAmount int,
- Description VARCHAR(200) NOT NULL,
- shoppingDate DateTime,
- CONSTRAINT [PK_ShoppingDetails] PRIMARY KEY CLUSTERED
- (
- [shopId] ASC
- )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
- ) ON [PRIMARY]
- select * from ShoppingDetails
Step 2 - Create ASP.NET Core Blazor Application
After installing all the prerequisites listed above and ASP.NET Core Blazor Language Services, click Start >> Programs >> Visual Studio 2017 >> Visual Studio 2017 on your desktop. Click New >> Project. Select Web >> ASP.NET Core Angular Web Application. Enter your project name and click OK.

Select Blazor (ASP.NET Core hosted) and click ok.

After creating ASP.NET Core Blazor Application, wait for few seconds. You will see the below structure in solution explorer.

What is new in ASP.NET Core Blazor solution?
When we will create our new ASP.NET Core Blazor application we can see as there will be 3 projects that will be automatically created in the Solution Explorer.
Client Project
The first project created is the Client project and it will be as our Solutionname.Client and here we can see our Solutionname as “BlazorASPCORE”. As the project named is a client and this project will be mainly focused for all the client-side view, here we will be adding all our page views to be displayed on the client side in the browser.

We can see a few of sample pages have been already added here and we can also see a shared folder like our MVC application where we will be having the Sharedfolder and Layout page for the Master page. Here in Blazor, we have the MainLayout which will work like the Master page and NavMenu for the left side menu display.
Server Project
As the name indicates, this project will be used as a Server project. This project is mainly used to create all our Controllers and WEB API Controllers to perform all business logic and perform CRUD operations using WEB-APIs. In our demo application, we will be adding a Web API in this Server project and all the WEB API in our Client application. This Server project will be getting/setting the data from the Database, and from our Client project we bind or send the result to this server to perform the CRUD operation in the database.

Shared Project
As the name is indicating, this project works like a shared project. This project works as a Model for our Server project and for the Client project. The Model declared in this Shared project will be used in both the Server and in the Client project. We will also install all the packages needed for our project here, for example, to use the Entity Framework we have to install all the packages in this Shared project.

Run to test the application
When we run the application, we can see that the left side has navigation and the right side contains the data. We can see as the default sample pages and menus will be displayed in our Blazor web site. We can use the pages or remove them and start with our own page.

Now, let’s see how to add a new page to make the online Shopping website.
Using Entity Framework
To use the Entity Framework in our Blazor application we need to install the below packages.
Install the Packages
Go to Tools and then select -> NuGet Package Manager -> Package Manager Console.

You can see the Console at the bottom of the VS 2017 IDE and in the right side of the combo box on the console select the Default project as your shared project” Select Shared”

- You can see the PM> and copy and paste the below line to install the Database Provider package. This package is used to set the database provider as SQL Server.
Install-Package Microsoft.EntityFrameworkCore.SqlServer
We can see as the package is installed in our Shared folder
Install the Entity Framework
- You can see the PM> and copy and paste the below line to install the EF package.
Install-Package Microsoft.EntityFrameworkCore.Tools
To Create DB Context and set the DB Connection string
- You can see the PM> and copy and paste the below line to set the Connection string and create DB Context. This is an important part as we give our SQL Server name, Database Name and SQL server UID and SQL Server Password to connect to our database to display our Shopping Cart details. We also give our both SQL Table name “ItemDetails, ShoppingDetails” to create the Model class in our Shared project.
- Scaffold-DbContext "Server= YourServerName;
- Database=ShoppingDB;
- userid=SQLID;
- password=SQLPWD;
- Trusted_Connection=True;
- MultipleActiveResultSets=true" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -Tables ItemDetails ,ShoppingDetails
Press enter create connection string, Model Class and Database Context.

We can see the ItemDetails and ShoppingDetails Model class and ShoppingDBContext class have been created in our Shared project. We will be using this Model and DBContext in our Server project to create our Web API.
Creating Web API for Getting Item details
To create our WEB API Controller, right-click trollers folder. Click Add New Controller.

Here, we will be using Scaffold method to create our WEB API. We select API Controller with actions, using Entity Framework.

Select our Model and DatabaseContext from the Shared project.

Select our ItemDetails Model from the Shared Project.

Select the Data Context Class as our ShoppingDBContext from the Shared project. Our Controller name will be automatically added, and if you need to you can change it and click the ADD.

We will be using only the Get method from our Web API.
Like this we also create a Web API for our ShoppingDetails.
In ShoppingDetails Web API controller get method we will be using the LINQ join query to select both Item and Shopping Details to be bound in home page to display the Item Details along with the shopping details to the users.
- [HttpGet]
- public IEnumerable<myShopping> GetShoppingDetails()
- {
- var results = (from items in _context.ItemDetails
- join shop in _context.ShoppingDetails
- on items.ItemId equals shop.ItemId
- select new myShopping
- {
- ShopId = shop.ShopId,
- ItemName = items.ItemName,
- ImageName = items.ImageName,
- UserName = shop.UserName,
- Qty = shop.Qty,
- TotalAmount = shop.TotalAmount,
- Description = shop.Description,
- ShoppingDate = shop.ShoppingDate
- }).ToList();
- return results;
- }
To test the Get Method, we can run our project and copy the GET method API path. Here, we can see our API path to get api/ItemDetails /
Run the program and paste API path to test our output.

For ShoppingDetails, we can get both the Itemdetails and ShoppingDetails table result. Here, we can see the result from Web API path /api/ ShoppingDetails

Now we will bind all these WEB API JSON results in our Razor View page from our Client project for displaying the Shopping List.
Working with Client Project
Note
In this article, we will create 2 Razor pages. In one Razor page, we will display the Item Details and users can do shopping by adding selected items to cart and save the shopping details to the database. In Homepage, we bind the shopping details of users with both Item and Shopping details.
Creating Shopping Page
Add Razor View
To add the Razor view page, right click the Pages folder from the Client project. Click on Add >> New Item

Select Razor View >> Enter your page name, here we have given the name as Shopping.chtml

In the Razor view page we have 3 parts of code, as the first is the import part where we import all the references and models for using in the view, HTML design, and data binding part; and finally, we have the function part to call all the web APIs to bind in our HTML page and also to perform client-side business logic to be displayed in View page.
Import part
First, we import all the needed support files and references in our Razor View page. Here, we have first imported our Model class to be used in our view and also imported HTTPClient for calling the Web API to bind the resultant JSON in our Razor page.
- @page "/Shopping"
- @using BlazorShoppingCarts.Shared
- @using BlazorShoppingCarts.Shared.Models
- @using Microsoft.AspNetCore.Blazor.Browser.Interop
- @using System.Collections.Generic
- @using Microsoft.AspNetCore.Blazor
- @inject HttpClient Http
Display All Items and Filter Items
Firstly we bind the Item details with Filter options and the user can add items for shopping by clicking on the Image.

Here, we bind all the Item Details.
HTML Part to Bind Item Details with Filter code
In the Init method, we get the ItemDetails from Web API and bind the result in an HTML table using a Foreach loop. We add the options as filter items from the Grid. For this we have added a new row in our table and added a textbox for filtering items for the ItemName. In the Filtering textbox onChange event, we pass the pressed key value to filter the Items. In Item List, we also bind the items with Image and in Image click, we add the selected item to Shopping Cart for shopping.
- @if (itmDetail == null)
- {
- <p><em>Loading...</em></p>
- }
- else
- {
- <table style="background-color:#FFFFFF; border: solid 2px #6D7B8D; padding: 5px;width: 100%;table-layout:fixed;" cellpadding="2" cellspacing="2">
- <tr style="height: 30px; background-color:#336699 ; color:#FFFFFF ;border: solid 1px #659EC7;">
- <td width="40" align="center">
- <b>Image</b>
- </td>
- <td width="40" align="center" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;cursor: pointer;">
- <b>Item Code</b>
- </td>
- <td width="120" align="center" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;cursor: pointer;">
- <b>Item Name</b>
- </td>
- <td width="120" align="center" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;cursor: pointer;">
- <b>Decription</b>
- </td>
- <td width="40" align="center" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;cursor: pointer;">
- <b>Price</b>
- </td>
- <td width="90" align="center" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;cursor: pointer;">
- <b>User Name</b>
- </td>
- </tr>
- <tr style="height: 30px; background-color:#336699 ; color:#FFFFFF ;border: solid 1px #659EC7;">
- <td width="40" align="center">
- Filter By ->
- </td>
- <td style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;">
- Item Name :
- </td>
- <td width="200" colspan="4" style="border: solid 1px #FFFFFF; padding: 5px;table-layout:fixed;">
- <input width="70" onchange=@OnItemNameChanged oninput="(this.dispatchEvent(new CustomEvent('change', {bubbles: true})))" />
- </td>
- </tr>
- <tbody>
- @foreach (var itmDtl in itmDetail)
- {
- <tr>
- <td align="center" style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- <img src="@itmDtl.ImageName" style="height:56px;width:56px" onclick="@(async () => await AddItemtoShoppingCart(@itmDtl))" />
- </span>
- </td>
- <td style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- @itmDtl.ItemId
- </span>
- </td>
- <td style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- @itmDtl.ItemName
- </span>
- </td>
- <td style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- @itmDtl.Description
- </span>
- </td>
- <td align="right" style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- @itmDtl.ItemPrice
- </span>
- </td>
- <td style="border: solid 1px #659EC7; padding: 5px;table-layout:fixed;">
- <span style="color:#9F000F">
- @itmDtl.AddedBy
- </span>
- </td>
- </tr>
- }
- </tbody>
- </table>
- }
Blazor Function part to bind the Item Details to HTML Grid with Filter functions
Function Part
Function part calls the web API to bind in our HTML page and also performs client-side business logic to be displayed in View page. In the function part, we first declare all the objects needed for our page.
- @functions {
- string UserName = "Shanu";
- string selectItemImage = "";
- string selectedItemName = "";
- int initialPrice = 0;
- string Messages = "";
- ShoppingDetails[] shoppingDetails;
- ItemDetails[] itmDetail;
- ShoppingDetails SD = new ShoppingDetails();
- ItemDetails ID = new ItemDetails();
- Boolean showAddtoCart = false;
Init Method
In the Init method, we get the result of Web API for ItemDetails and store it in the itmDetail object. We are using this object to be bound in our HTML table using the foreach statement.
In the Item Name column heading part, we have added a new row for performing the Filtering of the HTML grid. In the ItemName Column filter Textbox Change event, we pass the Textbox value. We call a common filtering method, FilteringList, and in this method, we pass the filtering column Textbox value and column Name to filter the ItemDetails and bind the result to HTML grid.
- protected override async Task OnInitAsync()
- {
- itmDetail = await Http.GetJsonAsync<ItemDetails[]>("/api/ItemDetails/");
- }
- // For Filtering by Item Name
- void OnItemNameChanged(UIChangeEventArgs args)
- {
- string values = args.Value.ToString();
- FilteringList(values);
- }
- //Filtering
- protected async Task FilteringList(String Value)
- {
- itmDetail = await Http.GetJsonAsync<ItemDetails[]>("/api/ItemDetails/");
- if (Value.Trim().Length > 0)
- {
- itmDetail = itmDetail.Where(x => x.ItemName.Contains(Value)).ToArray();
- }
- else
- {
- itmDetail = await Http.GetJsonAsync<ItemDetails[]>("/api/ItemDetails/");
- }
- }
Add Items to shopping cart

Users can add items to Shopping Cart by clicking on the Item Image from List. In the item image click we call the functions to bind the result to shopping cart for shopping. By default, we hide the shopping part from users and we set the showAddtoCart as false in page load and when the user clicks on the Item Image we set the showAddtoCart to true to display the Shopping details. Here is the HTML part to design the shopping part.
- @if (showAddtoCart == true)
- {
- <table style="background-color:#FFFFFF; border: dashed 3px #d55500; padding: 5px;width: 99%;table-layout:fixed;" cellpadding="2"
- cellspacing="6">
- <tr style="height: 30px; color:#336699 ;border: solid 1px #659EC7;">
- <td width="40px"> <img src="Images/shopping_cart64.png" height="38" width="38" /></td>
- <td>
- <h2> <strong>Add Items to Cart</strong></h2>
- </td>
- </tr>
- <tr>
- <td width="10px"> </td>
- <td>
- <table >
- <tr >
- <td style="width:250px;-moz-box-shadow: inset 0 0 5px #888;
- -webkit-box-shadow: inset 0 0 5px #888;
- box-shadow: inset 1px 1px 5px #888;
- display: block;">
- <img src="@selectItemImage" style="height:250px;width:250px" />
- </td>
- <td width="10"></td>
- <td align="left" width="500px" valign="top">
- <table style="width:500px;color:#9F000F;font-size:large;width:98%;" cellpadding="4" cellspacing="6">
- <tr style="height: 30px; color:#416048 ;border-bottom: solid 1px #659EC7;">
- <td>
- <h2>
- @selectedItemName
- </h2>
- </td>
- </tr>
- <tr style="height: 30px; color:#e13a19 ;border-bottom: solid 1px #659EC7;">
- <td>
- <h3>
- Price : @initialPrice
- </h3>
- </td>
- </tr>
- <tr style="height: 30px; color:#3a729a ;border-bottom: solid 1px #659EC7;">
- <td>
- <table>
- <tr>
- <td>
- <h3>
- Qty : @SD.Qty
- </h3>
- </td>
- <td>
- <table>
- <tr>
- <td>
- <img src="Images/UP.png" height="20" width="20" onclick="@IncreaseQty" />
- </td>
- </tr>
- <tr>
- <td>
- <img src="Images/Down.png" height="20" width="20" onclick="@DecreaseQty" />
- </td>
- </tr>
- </table>
- </td>
- <td style="color:#e13a19 ;">
- <h4>
- Total Amount : @SD.TotalAmount
- </h4>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- <tr style="height: 30px; color:#e13a19 ;">
- <td>
- <h3>
- Notes : <input type="text" class="form-control" bind="@SD.Description" />
- </h3>
- </td>
- </tr>
- <tr >
- <td>
- <h3>
- <button type="submit" class="btn btn-success" onclick="@(async () => await SaveShoppingetails())" style="width:220px;">
- <img src="Images/shopping_cart64.png" height="32" width="32" /> BUY NOW</button>
- </h3>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- </table>
- }
Item Image click event
When the user clicks on the Item Image from the list we call the AddItemtoShoppingCart and pass the selected ItemDetails to the function. In this function firstly, we set the showAddtoCart as true to show the Shopping list. We also bind the selected Item details to shoping list to add in Cart.
- protected async Task AddItemtoShoppingCart(ItemDetails itemAdd)
- {
- showAddtoCart = true;
- initialPrice = 0;
- SD.ItemId = itemAdd.ItemId;
- selectedItemName = itemAdd.ItemName;
- SD.Qty = 1;
- initialPrice = itemAdd.ItemPrice;
- SD.TotalAmount = itemAdd.ItemPrice;
- SD.UserName = UserName;
- selectItemImage = itemAdd.ImageName;
- SD.ShoppingDate = DateTime.Now;
- SD.Description = "";
- }
Shopping Cart Quantity Add and Reduce

In Shopping Cart user can increase /decrease the shopping Quantity and when user clicks on the up Image near Qty then we call the IncreaseQty method and in this method, we increment the Quantity and also update the Total Amount. It's the same as when a user clicks on the down icon. We call DecreaseQty method to do it in reverse order to decrement the quantity along with updating the Total Amount.
- void IncreaseQty()
- {
- if (SD.Qty > 0)
- {
- SD.Qty = SD.Qty + 1;
- SD.TotalAmount = initialPrice * SD.Qty;
- }
- }
- void DecreaseQty()
- {
- if (SD.Qty > 1)
- {
- SD.Qty = SD.Qty - 1;
- SD.TotalAmount = initialPrice * SD.Qty;
- }
- }
Save the Shopping List
Finally, when user clicks on the Buy Now button we pass the shopping details to save the result in our ShoppingDetails table in our database.
- //Save Shopping Details
- protected async Task SaveShoppingetails()
- {
- await Http.SendJsonAsync(HttpMethod.Post, "/api/ShoppingDetails/", SD);
- Messages = "Your Shopping is completed! Enjoy more shopping from my Site :)";
- SD = null;
- showAddtoCart = false;
- }
Shopping Order Items

In the home page we bind the user's shopping details with Item Name, Image, Order Date, Ordered Quantity and total amount. Users can also cancel the Order from the home page. When a user clicks on the Cancel Order we delete the shopping order details from the table.
- @if (myShopList == null)
- {
- <p><em>Loading...</em></p>
- }
- else
- {
- @foreach (var myShoppings in myShopList)
- {
- <table>
- <tr>
- <td>
- <table class="divContainer">
- <tr>
- <td align="left" width="112px">
- <span style="color:#9F000F">
- <img src="@myShoppings.ImageName" style="height:110px;width:110px" />
- </span>
- </td>
- <td align="left" valign="top">
- <table>
- <tr>
- <td valign="top">
- <h2 style="color:#244f2b">@myShoppings.ItemName </h2>
- </td>
- </tr>
- <tr>
- <td>
- Order Date : @myShoppings.ShoppingDate.Value.ToString("yyyy-MM-dd HH:mm")
- </td>
- </tr>
- <tr>
- <td>
- @myShoppings.Description
- </td>
- </tr>
- <tr>
- <td>
- <h3 style="color:#ff0000"> @myShoppings.TotalAmount (INR) / @myShoppings.Qty (QTY)</h3>
- </td>
- </tr>
- </table>
- </td>
- <td>
- <h3>
- <button type="submit" class="btn btn-danger" onclick="@(async () => await deleteConfirm(@myShoppings))" style="width:220px;">
- <img src="Images/delete.png" /> Cancel Order
- </button>
- </h3>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- <tr>
- <td style="height:10px;">
- </td>
- </tr>
- </table>
- }
- }
In the function part, first we declare all the object needed for this page and in Init method we load all the ShoppingDetails and bind the result in HTML grid.
- @functions {
- string UserName = "Shanu";
- ShoppingDetails SD = new ShoppingDetails();
- string Messages = "";
- myShopping[] myShopList;
- int shopID = 0;
- protected override async Task OnInitAsync()
- {
- shopID = 0;
- myShopList = await Http.GetJsonAsync<myShopping[]>("/api/ShoppingDetails/");
- }
Cancel Order

When users click on the Cancel Order button we call the deleteConfirm method and pass the selected ShoppingDetails ID for deletion. Before deletion we get the conformation message as “Are you Sure to Cancel your Item order “ .
- @if (Messages != "")
- {
- <div id="outPopUp" style="position: absolute; width: 552px; height: 320px; z-index: 15; top: 38%; left: 50%; margin: -100px 0 0 -150px; background: #fbde5a;">
- <div id="outPopUp" style="position: absolute; width: 551px; height: 248px; background: #fc3a2e;">
- <img src="Images/C1.png" onclick="@closeMessage" />
- <span style="color:#ECF3F4;font-size:xx-large;display: inline-block; margin: 10px 10px 0 0; padding: 5px 10px ">
- @Messages
- </span>
- </div>
- <div id="outPopUp" style="position: absolute; top:260px; width:350px;left:24%; height: 70px; ">
- <h3>
- <button type="submit" class="btn btn-success" onclick="@(async () => await deleteShopping())" style="width:150px;">
- <img src="Images/tick.png" height="32px" width="32px"/> Ok
- </button>
- <button type="submit" class="btn btn-danger" onclick="@closeMessage" style="width:150px;">
- <img src="Images/delete1.png" height="32px" width="32px"/> Cancel
- </button>
- </h3>
- </div>
- </div>
- }
- protected async Task deleteConfirm(myShopping sds)
- {
- shopID = sds.ShopId;
- Messages = "Are you Sure to Cancel your " + sds.ItemName + " Order ?";
- }
If user clicks on ok button we call the deleteShopping method and in this method, we delete the ShoppingDetails by the selected Shopping ID. If a user clicks on the Cancel button then we hide the Confirmation message and cancel deleting the Shopping order.
- protected async Task deleteShopping()
- {
- await Http.DeleteAsync("/api/ShoppingDetails/" + Convert.ToInt32(shopID));
- Messages = "";
- myShopList = await Http.GetJsonAsync<myShopping[]>("/api/ShoppingDetails/");
- }
- void closeMessage()
- {
- shopID = 0;
- Messages = "";
- }
Navigation Menu
Now we need to add this newly added Shopping Razor page to our left Navigation. For adding this, open the Shared Folder and open the NavMenu.cshtml page, and add the menu
- <li class="nav-item px-3">
- <NavLink class="nav-link" href="" Match=NavLinkMatch.All>
- <span class="oi oi-home" aria-hidden="true"></span> Home
- </NavLink>
- </li>
- <li class="nav-item px-3">
- <NavLink class="nav-link" href="Shopping">
- <img src="Images/shopping_cart64.png" height="32" width="32" /> Let's Shop
- </NavLink>
- </li>
Conclusion
When creating the DBContext and setting the connection string, don’t forget to add your SQL connection string. I hope you like this article, and in the next article we will see more examples to work with Blazor. It's really very cool and awesome to work with Blazor.